Over time I have written many PowerShell functions where I have needed to implement calls to WMI or CIM classes. Usually, these classes have many properties in the resultset and each property has its own datatype.
The problem for me was to find out about these properties and data types and I would like to show you the function that I use for that purpose written as an Add-on in PowerShell ISE.
Let me quickly demonstrate the function with one simple example. We call the function and pass the CIM class name as parameter ( CIM_Processor CIM class name in this example ).
Get-CIMClassProperty -classname "CIM_Processor"
As a result, presented in PowerShell grid, we get all the properties and their data types which we can get in the resultset when we call CIM_Processor class using Get-CimInstance CmdLet.
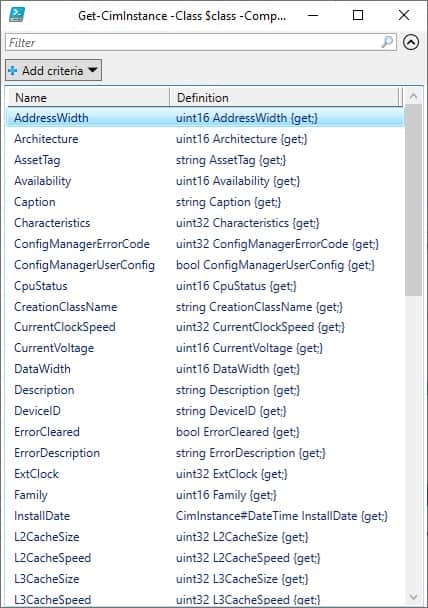
INFO: If you want to have a select statement with all the property names listed for the class please read this article where I have written the second Add-On function that does just that. How To Write Select Statement For All Properties Of CIM Or WMI Class With PowerShell.
Table of Contents
Get-CIMClassProperty CmdLet – Explained
This CmdLet covers not just CIM classes but rather WMI classes as well.
Get-CIMClassProperty CmdLet – Input Parameters
As input parameters we have:
- classname – Name of the CIM or WMI class that can be passed directly calling the function.
- SelectedText – Value of the selected text in the PowerShell ISE Script Pane. Write the class name and select it in PowerShell ISE Script Pane and click on menu item Add-ons -> “Get CMI Class Properties” to call the same function.
- InstallMenu – a parameter used to add function as a menu item in Add-ons menu of PowerShell ISE if set to true.
- ComputerName – Name of computer with a default value of local machine.
Function Get-CIMClassProperty {
[CmdletBinding()]
param (
[Parameter(HelpMessage="CMI class name.")]
[string]$classname,
$SelectedText = $psISE.CurrentFile.Editor.SelectedText,
$InstallMenu,
$ComputerName = 'localhost'
)
}
INFO: To know more about PowerShell Parameters and Parameter Sets with some awesome examples please read the following articles How To Create Parameters In PowerShell and How To Use Parameter Sets In PowerShell Functions.
BEGIN Block
In the BEGIN block we:
- If parameter $InstallMenu set to true we add the function as a menu item to Add-ons menu of PowerShell ISE environment.
BEGIN {
if ($InstallMenu)
{
Write-Verbose "Try to install the menu item, and error out if there's an issue."
try
{
$psISE.CurrentPowerShellTab.AddOnsMenu.SubMenus.Add("Get CMI Class Properties",{Get-CIMClassProperty},"Ctrl+Alt+P") | Out-Null
}
catch
{
Return $Error[0].Exception
}
}
}
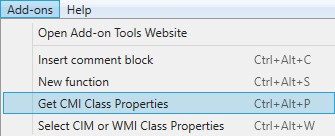
PROCESS Block
In the PROCESS block we:
- We run this piece of code only if the function has been added as a menu item (!$InstallMenu)
- Error Handling using try-catch blocks and writing errors in an external text file using Write-ErrorLog CmdLet.
- Prepare class name depending on which input parameter was provided.
- We get properties using the Get-CimInstance CmdLet and pipeline the result to the standard PowerShell grid.
PROCESS {
if (!$InstallMenu)
{
Write-Verbose "Don't run a function if we're installing the menu"
try
{
Write-Verbose "Return all the properties for CMI Class."
if ($SelectedText )
{
$class = "$SelectedText"
} else {
$class = "$classname"
}
Get-CimInstance -Class $class -ComputerName $ComputerName | Get-Member -MemberType Property | Select-Object name, definition | Out-GridView
}
catch
{
Return $Error[0].Exception
}
}
}
INFO: To learn about PowerShell Error Handling and code debugging please read the following articles: How To Log PowerShell Errors And Much More and How To Debug PowerShell Scripts.
INFO: PowerShell Pipelining is a very important concept and I highly recommend you to read the article written on the subject. How PowerShell Pipeline Works. Here I have shown in many examples the real power of PowerShell using the Pipelining.
END Block
END block is empty.
INFO: To understand BEGIN, PROCESS and END blocks in PowerShell please read PowerShell Function Begin Process End Blocks Explained With Examples.
Comment-Based Help Section
For every one of my own CmdLets, I write Comment-Based help as well.
INFO: If you want to learn how to write comment-based Help for your own PowerShell Functions and Scripts please read these articles How To Write PowerShell Help (Step by Step). In this article How To Write PowerShell Function’s Or CmdLet’s Help (Fast), I explain the PowerShell Add-on that helps us be fast with writing help content.
Execution Examples Region
In order to make this function PowerShell ISE Add-on we need to call the function for the first time with parameter InstallMenu set to true.
In the next section, I explain how to set up function, module file, module manifest file and profile file in PowerShell to achieve that function becomes part of the PowerShell ISE environment.
#region Execution examples
Get-CIMClassProperty -InstallMenu $true
#Get-CIMClassProperty -classname "CIM_Processor"
#endregion
How To Add Get-CIMClassProperty CmdLet As Add-On Into PowerShell ISE
Here are the steps that we have followed in order to have that Add-on in PowerShell ISE environment: ( You have all this already done if you have downloaded the zip file with the source code.)
- have created 09addons folder where my Modules are and for me, that was this location C:\Users\$env:USERNAME\[My] Documents\WindowsPowerShell\Modules
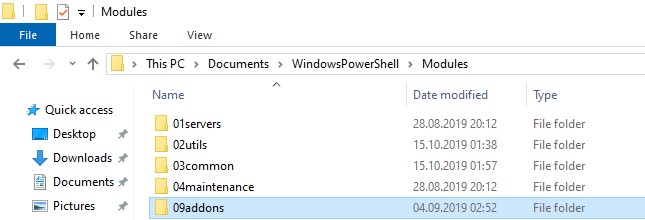
- have saved Get-CIMClassProperty as PowerShell script (.ps1 file) in 09addons folder.

- have customized 09addons.psm1 module file with this line of code in order to load Get-CIMClassProperty CmdLet into 09addons module.
. $psScriptRoot\GetCIMClassProperty.ps1
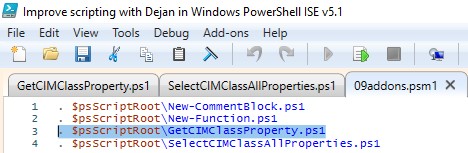
- have customized Manifest file 09addons.psd1 to export the newly created function Get-CIMClassProperty.

- have customized Profile file ( Microsoft.PowerShellISE_profile.ps1) location for me: (C:\Users\$env:USERNAME\[My] Documents\WindowsPowerShell) with this line of code in order to Import 09addons Module.
Import-Module 09addons
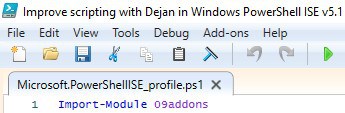
INFO: If you want to learn more about PowerShell Profiles and Modules please read the following articles. How To Create A Powershell Module (Different Approach) and How To Create PowerShell Profile Step by Step with Examples.
INFO: Interested in PowerShell Module Manifest please read How To Create A PowerShell Module Manifest section.
What Happens When You Start PowerShell ISE?
If you wonder why we have done all the steps in the previous section here is an explanation of PowerShell Workflow when every session starts
When you open Windows PowerShell ISE here are things that happen in the background even before we see the ISE window:
- New PowerShell session starts for the current user
- PowerShell profile files have been loaded into the session (one of them is Microsoft.PowerShellISE_profile.ps1 that we have just customized)
- The profile file will import modules. In our example, it will load the 09addons module among the others
- When the modules are imported the code inside the Script module file (.psm1) is executed. In our example, GetCIMClassProperty.ps1 script file will be executed
- When a script file is executed Get-CIMClassProperty CmdLet function is loaded into the session. In our example this code will be executed:
Get-CIMClassProperty -InstallMenu $true
… and that will add Get-CIMClassProperty as Add-On Menu Item in Windows PowerShell ISE so we can use it as we need it. - Finally, our environment is customized and we see Windows PowerShell ISE application with Add-on “Get CMI Class Properties” added into our environment and we can start working.
I hope this explains the steps in the previous section.
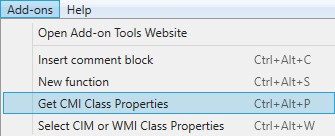
How To Use Get-CIMClassProperty CmdLet
We have several ways to exploit this CmdLet.
IMPORTANT: Open new PowerShell ISE Script Pane in order to call Get-CIMClassProperty CmdLet.
- We can call Get-CIMClassProperty CmdLet providing the name of CIM or WMI class in new PowerShell ISE Script Pane and run the script with pressing F5.
Get-CIMClassProperty -classname "CIM_Processor"

- We can write just the name of CIM or WMI class, SELECT the name that we have just written and go to the Add-ons menu and click on “Get CMI Class Properties”.
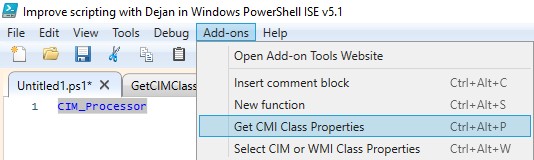
- We can write just the name of CIM or WMI class like in the previous example, SELECT the name that we have just written and press the shortcut Ctrl + Alt + P to run the same menu item “Get CMI Class Properties”.
The result will be the same for all of them as explained at the beginning of this article and the result is presented as grid.
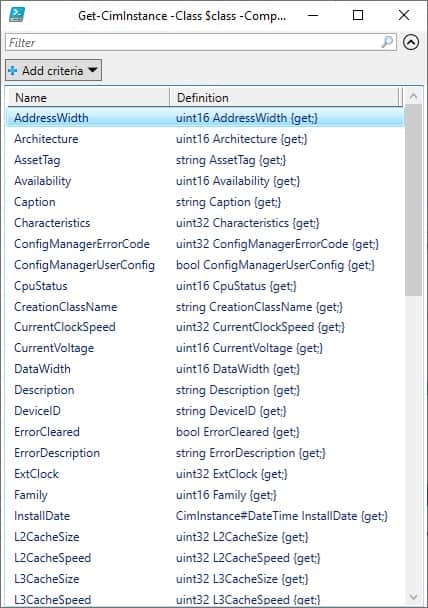
Get-CIMClassProperty CmdLet Code
INFO: My best advice to every PowerShell scripter is to learn writing own PowerShell Advanced Functions and CmdLets and I have written several articles explaining this, so please read them. How To Create A Custom PowerShell CmdLet (Step By Step). Here I explain how to use PowerShell Add-on Function to be faster in writing PowerShell Functions How To Write Advanced Functions Or CmdLets With PowerShell (Fast).
Here is the source code of the whole Get-CIMClassProperty Add-on CmdLet. Enjoy!
<#
.SYNOPSIS
Get all the properties for CIM (Common Information Model) or WMI (Windows Management Instrumentation) class.
.DESCRIPTION
Get all the properties for CIM (Common Information Model) or WMI (Windows Management Instrumentation) class. This is usefull when one wants to know what can be output from CIM or WMI class.
Which properties might be usefull and which are not.
.PARAMETER classname
CIM or WMI class name e.g. Win32_OperatingSystem
.EXAMPLE
Get-CIMClassProperty -classname "Win32_OperatingSystem"
.EXAMPLE
Get-CIMClassProperty -classname "CIM_Processor"
.NOTES
FunctionName : Get-CIMClassProperty
Created by : Dejan Mladenovic
Date Coded : 01/18/2020 04:34:41
More info : http://improvescripting.com/
.LINK
How To List CIM Or WMI Class All Properties And Their Datatypes With PowerShell
.OUTPUT
Output GridView
#>
Function Get-CIMClassProperty {
[CmdletBinding()]
param (
[Parameter(HelpMessage="CMI class name.")]
[string]$classname,
$SelectedText = $psISE.CurrentFile.Editor.SelectedText,
$InstallMenu,
$ComputerName = 'localhost'
)
BEGIN {
if ($InstallMenu)
{
Write-Verbose "Try to install the menu item, and error out if there's an issue."
try
{
$psISE.CurrentPowerShellTab.AddOnsMenu.SubMenus.Add("Get CMI Class Properties",{Get-CIMClassProperty},"Ctrl+Alt+P") | Out-Null
}
catch
{
Return $Error[0].Exception
}
}
}
PROCESS {
if (!$InstallMenu)
{
Write-Verbose "Don't run a function if we're installing the menu"
try
{
Write-Verbose "Return all the properties for CMI Class."
if ($SelectedText )
{
$class = "$SelectedText"
} else {
$class = "$classname"
}
Get-CimInstance -Class $class -ComputerName $ComputerName | Get-Member -MemberType Property | Select-Object name, definition | Out-GridView
}
catch
{
Return $Error[0].Exception
}
}
}
END { }
}
#region Execution examples
Get-CIMClassProperty -InstallMenu $true
#Get-CIMClassProperty -classname "CIM_Processor"
#endregion
Well done! REMEMBER – PowerShell script every day!